Sentinel Controlled While Loop C++
Software Design Using C++
- A sentinel value is a special value used to terminate a loop when reading data. In the following program, test scores are provided (via user input). Once the sentinel value of -1 is input, the loop terminates. At that point, the average of the test scores will be printed.
- Sentinel controlled while loop C Tutorial. Control Structures - while loop - do-while loop - for loop - Goto - break - continue statements - Duration: 18:16. EzEd Channel 27,359 views.
- In computer programming, a sentinel value (also referred to as a flag value, trip value, rogue value, signal value, or dummy data) is a special value in the context of an algorithm which uses its presence as a condition of termination, typically in a loop or recursive algorithm. The sentinel value is a form of in-band data that makes it possible to detect the end of the data when no out-of.
C Programming Tutorials brought to you by TONY TUTORIALS. Sentinel-controlled loop with a String variable condition. Ranch Hand Posts: 76. I have gone over and over the textbook sections that deal with loops, sentinel-controlled loops, while loops, do.while loops, for loops, if.else loops, nested loops.
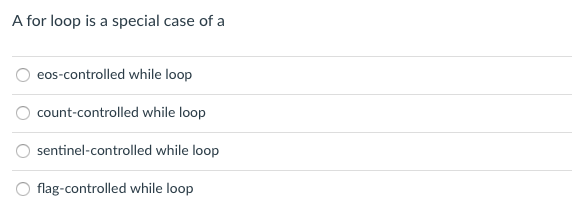
Programming Patterns
A lot of programming is based on using existing patterns. This section presents a number of simple, but commonly used, programming patterns. If you are working on a problem that fits one of these, pull it out and use it, adapting it as needed. This is usually much faster than trying to invent something new.
Skip Guard Plan
Often one needs to skip over a section of code when certain conditions arise. For example, you may need to skip over a computation when it would result in an attempt to divide by zero:
In general, the pattern is given by the following outline, which is written in a mixture of English and C++.
Count-Controlled Loop Plan

Process
function, etc. Input Checking Plan
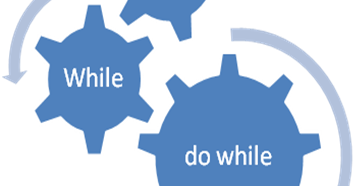
Unacceptable
function shown below might, of course, be replaced by a simple condition. There is no need to write a function here unless the algorithm for determining unacceptable items is fairly complex. Sentinel-Controlled Loop Plan
Here the idea is to enter a special item (the sentinel) to indicate end of the sequence of data items to be processed. The sentinel itself is not one of the data items. One might, for example, have data consisting of a string of positive integers and use 0 to signal the end of the data. Or, one might tell the user to press CTRL z to indicate the end of the data. (In Linux one uses CTRL d to end data input, as CTRL z is used to suspend the current process.) This pattern uses EOF (end of file) as a sentinel. The outline is as follows:
As an example, let's write out more detail of how to use EOF as a sentinel. Suppose we want to enter and process a sequence of integers, with CTRL z used to indicate the end of data entry. Then we would use something like this:
The fail
function returns true whenever the previous stream operation failed to input an acceptable value. The usual reasons for such failure are that the user pressed CTRL z or that the user entered something (such as abcd
) that could not be interpreted as an integer. Although the code shown above reads data from the keyboard, it could easily be adapted to read the data from a file.
The most general form of this pattern is as follows. Again, we use part English and part C++.
Do a Problem Until the User Wants to Quit
Often you want to allow the user to do some task repeatedly. A simple way to allow this is to ask the user whether or not to do another of whatever the task at hand might be.
Menu-Driven Plan
This is similar to the previous plan, but adds a menu that is printed to show the user possible commands, here assumed to be the letters a, b, c, and q. The letter q is used as the signal that the user wants to quit. Of course, you can use integers or whatever is convenient. After getting the user's choice the plan picks out the proper task to execute. Then, as long as the choice is not to quit, loop around and present the menu again, etc.
Adding a Counter to a Sentinel-Controlled While Loop
We have already seen how to create a sentinel-controlled while loop. This type of loop is used to read in data until the sentinel is reached. Often we want to count the number of data items read in (not including the sentinel itself). All you need is an integer
Count
variable that is initialized to zero and incremented each time a new data item is obtained. Here is how this is done: Adding a Running Total to a Sentinel-Controlled While Loop
We can also add a running total to a sentinel-controlled while loop. The following example finds the total of all of the data items entered. Of course, the data items must be of a type that can be added, perhaps integers or floats, and the variable
Total
must have the same type. This example assumes that finding the total is the only processing that is needed on the data items. Finding an Average in a Sentinel-Controlled While Loop
Since we know how to total and count the data items read in by a sentinel-controlled while loop, we can now easily find the average of these data items.
Flag Controlled Loop
Related Items
Back to the main page for Software Design Using C++Last updated: August 27, 2009
Disclaimer
Saint Vincent College - Computing and Information Systems Department
300 Fraser Purchase Road • Latrobe, PA 15650
In computer programming, a sentinel value (also referred to as a flag value, trip value, rogue value, signal value, or dummy data)[1] is a special value in the context of an algorithm which uses its presence as a condition of termination, typically in a loop or recursive algorithm.
The sentinel value is a form of in-band data that makes it possible to detect the end of the data when no out-of-band data (such as an explicit size indication) is provided. The value should be selected in such a way that it is guaranteed to be distinct from all legal data values since otherwise, the presence of such values would prematurely signal the end of the data (the semipredicate problem). A sentinel value is sometimes known as an 'Elephant in Cairo,' due to a joke where this is used as a physical sentinel. In safe languages, most sentinel values could be replaced with option types, which enforce explicit handling of the exceptional case.
Examples[edit]
Some examples of common sentinel values and their uses:
- Null character for indicating the end of a null-terminated string
- Null pointer for indicating the end of a linked list or a tree.
- A set most significant bit in a stream of equally spaced data values, for example, a set 8th bit in a stream of 7-bit ASCII characters stored in 8-bit bytes indicating a special property (like inverse video, boldface or italics) or the end of the stream
- A negative integer for indicating the end of a sequence of non-negative integers
Variants[edit]

A related practice, used in slightly different circumstances, is to place some specific value at the end of the data, in order to avoid the need for an explicit test for termination in some processing loop, because the value will trigger termination by the tests already present for other reasons. Unlike the above uses, this is not how the data is naturally stored or processed, but is instead an optimization, compared to the straightforward algorithm that checks for termination. This is typically used in searching.[2][3]
For instance, when searching for a particular value in an unsorted list, every element will be compared against this value, with the loop terminating when equality is found; however, to deal with the case that the value should be absent, one must also test after each step for having completed the search unsuccessfully. By appending the value searched for to the end of the list, an unsuccessful search is no longer possible, and no explicit termination test is required in the inner loop; afterward, one must still decide whether a true match was found, but this test needs to be performed only once rather than at each iteration.[4]Knuth calls the value so placed at the end of the data, a dummy value rather than a sentinel.
Examples[edit]
Array[edit]
For example, if searching for a value in an array in C, a straightforward implementation is as follows; note the use of a negative number (invalid index) to solve the semipredicate problem of returning 'no result':
However, this does two tests at each iteration of the loop: whether the value has been found and whether the end of the array has been reached. This latter test is what is avoided by using a sentinel value. Assuming the array can be extended by one element (without memory allocation or cleanup; this is more realistic for a linked list, as below), this can be rewritten as:
The test for i < len
is still present, but it has been moved outside the loop, which now contains only a single test (for the value), and is guaranteed to terminate due to the sentinel value. There is a single check on termination if the sentinel value has been hit, which replaces a test for each iteration.
It is also possible to temporarily replace the last element of the array by a sentinel and handle it, especially if it is reached:
See also[edit]
References[edit]
- ^Knuth, Donald (1973). The Art of Computer Programming, Volume 1: Fundamental Algorithms (second edition). Addison-Wesley. pp. 213–214, also p. 631. ISBN0-201-03809-9.
- ^Mehlhorn, Kurt; Sanders, Peter (2008). Algorithms and Data Structures: The Basic Toolbox 3 Representing Sequences by Arrays and Linked Lists(PDF). Springer. ISBN978-3-540-77977-3. p. 63
- ^McConnell, Steve (2004). Code Complete (2nd ed.). Redmond: Microsoft Press. p. 621. ISBN0-7356-1967-0.
- ^Knuth, Donald (1973). The Art of Computer Programming, Volume 3: Sorting and searching. Addison-Wesley. p. 395. ISBN0-201-03803-X.
Sentinel Controlled While Loop C++ Loop
